Lately I’ve gotten more and more interested in building out applications to address pain points on Square’s platform. The challenge I run into for every project though is navigating the OAuth flow and handling user credentials. I’ve had great experiences using Auth0 in the past for handling these issues on other platforms, but have never quite been able to get it to work with Square.
I spent some time trying to get a better handle on the custom social login flow in Auth0 and was finally able to get and Auth0 OAuth flow working with Square. The first step is to navigate to Authentication > Social in Auth0 and click ‘Create Connection’
The next step is to navigate to your Square Applications page in the Developer Dashboard and open your app or create a new one. From your application page you should see an OAuth section in the navigation bar on the left. You will want to retrieve your Production Application ID and Application secret for the next step.
You must also set your Callback URL to ’https://[YOUR_AUTH0_USERNAME].auth0.com/login/callback’
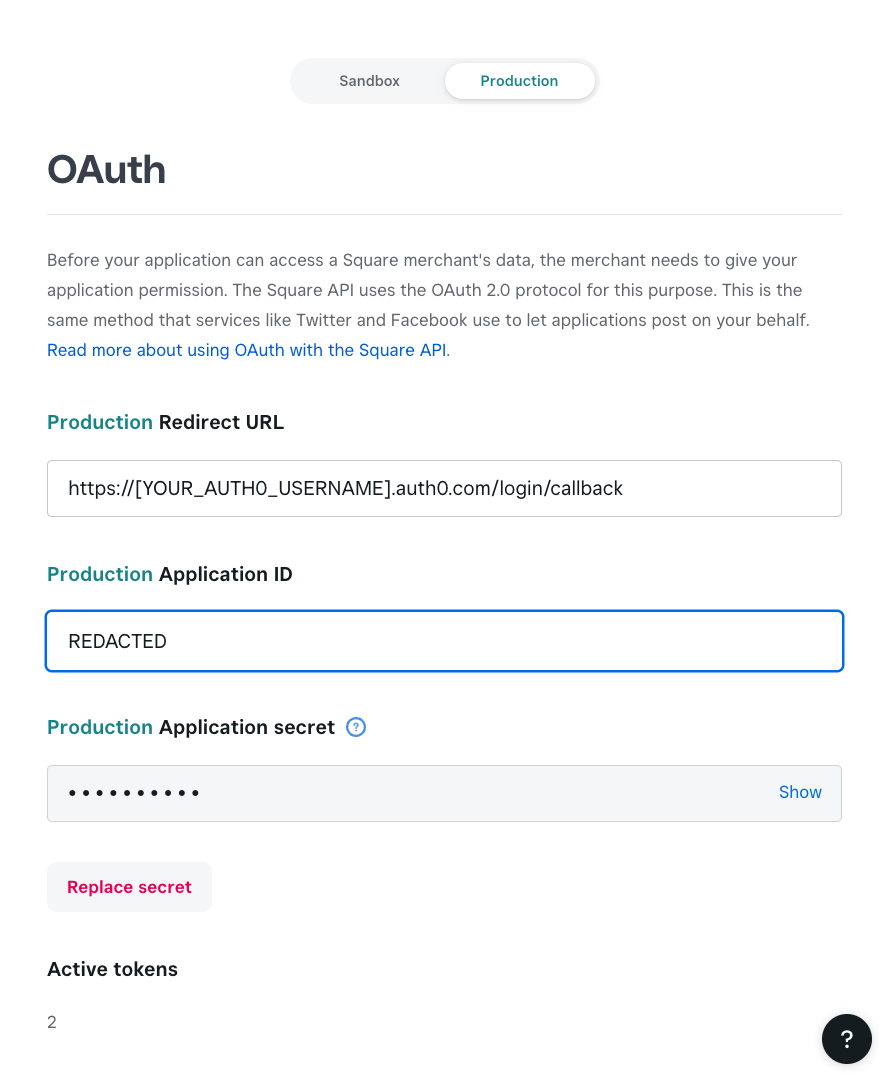
Navigate back to Auth0 and add the following settings:
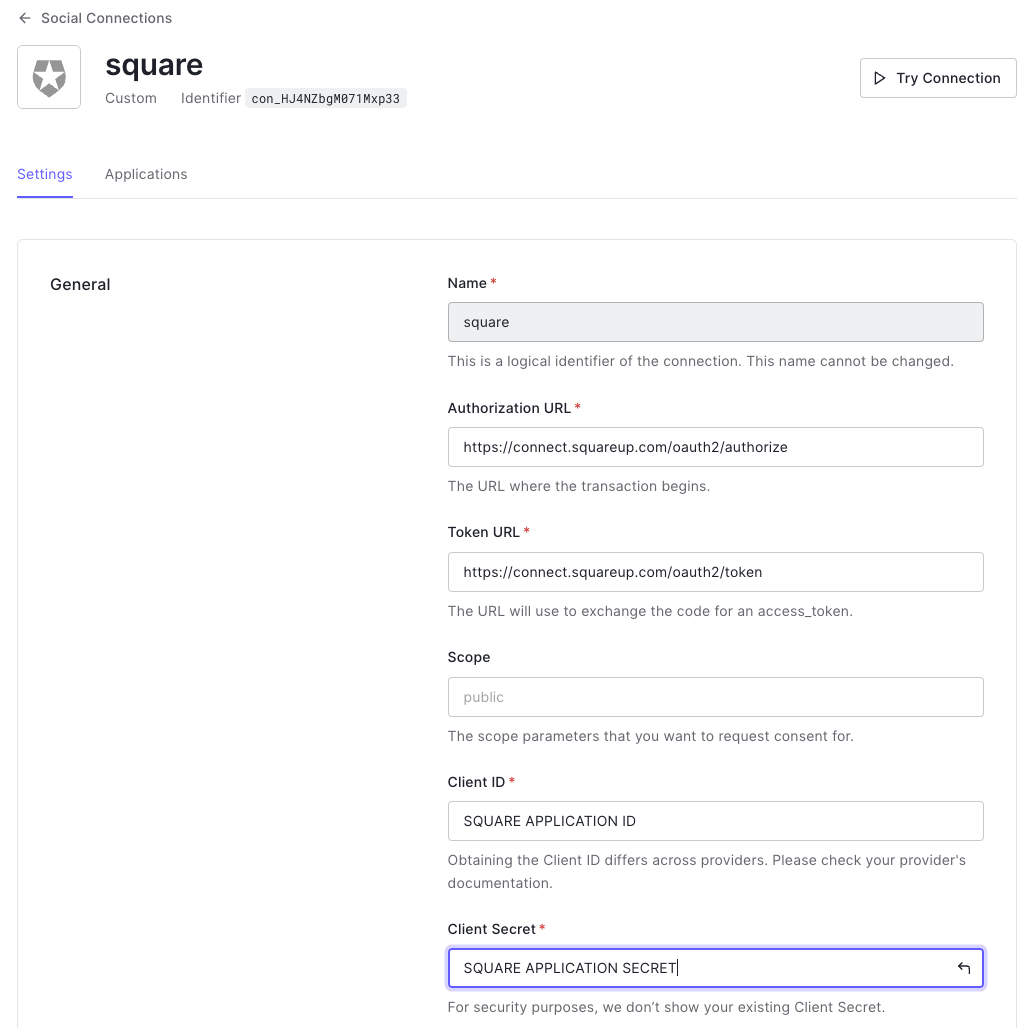
The last part is adding a Fetch User Profile Script that will build the user profile for the logged in merchant. Here is what I used:
function(accessToken, ctx, cb) {
request({
method: 'GET',
url:'https://connect.squareup.com/v2/merchants',
headers: {
Authorization: 'Bearer ' + accessToken
}
}, function(err, resp, body) {
if(err) {
return cb(err);
}
if(resp.statusCode !== 200) {
return cb(new Error('StatusCode:' + resp.statusCode));
}
let bodyParsed;
try {
bodyParsed = JSON.parse(body);
} catch (jsonError) {
return cb(new Error(body));
}
const merchant = bodyParsed.merchant[0];
let profile = {
user_id: merchant.id,
given_name: merchant.business_name,
name: merchant.business_name,
};
cb(null, profile);
});
}
At this point you should be good to go. Click ‘Try Connection’ at the top of the page and you should see a page with ‘It Works!’ at the top. At this point you should have a new user in Auth0 with an attached Square identity. You can fetch the details of this user through the Auth0 Get User (api/v2/users) endpoint and extract the access token for the user.